A good reference of creating sequence and using it can be found at
https://docs.mongodb.org/manual/tutorial/create-an-auto-incrementing-field/
This can be achieved in Mongoose,
1) Step 1
From mongo shell create the counter collection as below
db.counters.insert({_id: "userid",seq: 0})
2)
create a counter schema as below (counter.js )
var mongoose = require('mongoose');
var counterSchema = {
_id: {type: String, required: true},
seq: { type: Number, default: 0 }
};
module.exports = mongoose.model('Counter', counterSchema);
3) Use it (for example -- UserHandler.js)
var mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/cchPerfApp');
var User = require('../model/user');
var counter = require('../model/counter');
var saveRequest = function() {
counter.findByIdAndUpdate({_id: 'userid'}, {$inc: { seq: 1} }, function(error, counter) {
if(error) {
console.log(error)
}else {
var user = new User();
user._id = counter.seq;
user.name = "xyz"
user.save(function(err){
}
}
}
}
JyGnash
Wednesday, February 3, 2016
Monday, December 8, 2014
Installing Node-Oracle on Windows 7
Assuming that you Nodejs installed and working on your local pc.
Software Required
Setup
Step 1
Install Windows 7 SDK
1) Uninstall any c++ redistributable you have on your pc.
2) If you run in to problem installing the windows 7 sdk , try doing what is provided at this help (http://ctrlf5.net/?p=184)
Step 2
Install Visual C++ 2010 Express
Step 3
Download and install Python
Step 4
Download the Oracle Basic + SDK. (kindly download 64 bit /32 bit depending on your OS)
Extract it at one place
C:\oracle\instantclient_12_1_64
Step 5
Download the node-oracle zip and extract it at
C:\oracle\node-oracle-master
Step 6
set the following variable or create a bat file that you can run.
set OCI_LIB_DIR=C:\oracle\instantclient_12_1_64\sdk\lib\msvc\vc10
set OCI_INCLUDE_DIR=C:\oracle\instantclient_12_1_64\sdk\include
set OCI_VERSION=12
set NLS_LANG=.UTF8 # Optional, but required to support international characters
Path=%PATH%;C:\oracle\instantclient_12_1_64\vc10;C:\oracle\instantclient_12_1_64;C:\Users\jignesh.rangwala\AppData\Roaming\npm;C:\Python27\python.exe;C:\Python27\pythonw.exe
Step 7
Launch the Windows SDK 7.1 Command Prompt, go to the directory of your node-oracle module (C:\oracle\node-oracle-master)
If any where in this step you get file permission errors open the Windows SDK 7.1 Command Prompt as Administrator
1) run npm install -g
2) If everything runs correctly this will build and create node oracle modules at
C:\Users\[user]\AppData\Roaming\npm\node_modules
3) If you not defined yet , define an enviroment variable
NODE_PATH=C:\Users\[user]\AppData\Roaming\npm\node_modules
Run a sample testoracle.js
------------------------------------------------------------------
var oracle = require('oracle'); //without tns var connString = "(DESCRIPTION=(ADDRESS=(PROTOCOL=TCP)(HOST=localhost)(PORT=1521))(CONNECT_DATA=(SERVER=DEDICATED)(SERVICE_NAME=db)))"; var connectData = { "tns": connString, "user": "user", "password": "pwd" }; oracle.connect(connectData, function(err, connection) { if (err) { console.log("Error connecting to db:", err); return; } connection.execute("SELECT systimestamp FROM dual", [], function(err, results) { if (err) { console.log("Error executing query:", err); return; } console.log(results); connection.close(); // call only when query is finished executing }); });
Software Required
- Windows 7.1 SDK (Microsoft Windows SDK for Windows 7 and .NET Framework 4.0)
http://www.microsoft.com/en-us/download/details.aspx?id=8279 - Visual C++ 2010 Express
http://www.visualstudio.com/en-us/downloads#d-2010-express - Python 2.7
https://www.python.org/downloads/ - Oracle Download
Instant Client Package - Basic: All files required to run OCI, OCCI, and JDBC-OCI applications ( instantclient-basic-windows.x64-12.1.0.2.0)
*Instant Client Package - SDK: Additional header files and an example makefile for developing Oracle applications with Instant Client (instantclient-sdk-windows.x64-12.1.0.2.0)
http://www.oracle.com/technetwork/topics/winx64soft-089540.html - Node package For Oracle
https://github.com/joeferner/node-oracle
Setup
Step 1
Install Windows 7 SDK
1) Uninstall any c++ redistributable you have on your pc.
2) If you run in to problem installing the windows 7 sdk , try doing what is provided at this help (http://ctrlf5.net/?p=184)
Step 2
Install Visual C++ 2010 Express
Step 3
Download and install Python
Step 4
Download the Oracle Basic + SDK. (kindly download 64 bit /32 bit depending on your OS)
Extract it at one place
C:\oracle\instantclient_12_1_64
Step 5
Download the node-oracle zip and extract it at
C:\oracle\node-oracle-master
Step 6
set the following variable or create a bat file that you can run.
set OCI_LIB_DIR=C:\oracle\instantclient_12_1_64\sdk\lib\msvc\vc10
set OCI_INCLUDE_DIR=C:\oracle\instantclient_12_1_64\sdk\include
set OCI_VERSION=12
set NLS_LANG=.UTF8 # Optional, but required to support international characters
Path=%PATH%;C:\oracle\instantclient_12_1_64\vc10;C:\oracle\instantclient_12_1_64;C:\Users\jignesh.rangwala\AppData\Roaming\npm;C:\Python27\python.exe;C:\Python27\pythonw.exe
Step 7
Launch the Windows SDK 7.1 Command Prompt, go to the directory of your node-oracle module (C:\oracle\node-oracle-master)
If any where in this step you get file permission errors open the Windows SDK 7.1 Command Prompt as Administrator
1) run npm install -g
2) If everything runs correctly this will build and create node oracle modules at
C:\Users\[user]\AppData\Roaming\npm\node_modules
3) If you not defined yet , define an enviroment variable
NODE_PATH=C:\Users\[user]\AppData\Roaming\npm\node_modules
Run a sample testoracle.js
------------------------------------------------------------------
var oracle = require('oracle'); //without tns var connString = "(DESCRIPTION=(ADDRESS=(PROTOCOL=TCP)(HOST=localhost)(PORT=1521))(CONNECT_DATA=(SERVER=DEDICATED)(SERVICE_NAME=db)))"; var connectData = { "tns": connString, "user": "user", "password": "pwd" }; oracle.connect(connectData, function(err, connection) { if (err) { console.log("Error connecting to db:", err); return; } connection.execute("SELECT systimestamp FROM dual", [], function(err, results) { if (err) { console.log("Error executing query:", err); return; } console.log(results); connection.close(); // call only when query is finished executing }); });
------------------------------------------------------------------
If you get this error,
fs.js:438
return binding.open(pathModule._makeLong(path), stringToFlags(flags), mode); ^
Error: ENOENT, no such file or directory 'C:\oracle\node-oracle-master\test\tests-settings.json'
while running your program, just make sure your path should have
C:\oracle\instantclient_12_1_64\sdk\lib\msvc\vc10;
C:\oracle\instantclient_12_1_64;
before C:\oracle\product\11.2.0\client_1\bin
ie your path should be like this
C:\oracle\instantclient_12_1_64\sdk\lib\msvc\vc10;C:\oracle\instantclient_12_1_64;C:\oracle\product\11.2.0\client_1\bin
Just Verified the same softwares/steps works on windows server 2008 r2
If you get this error,
fs.js:438
return binding.open(pathModule._makeLong(path), stringToFlags(flags), mode); ^
Error: ENOENT, no such file or directory 'C:\oracle\node-oracle-master\test\tests-settings.json'
while running your program, just make sure your path should have
C:\oracle\instantclient_12_1_64\sdk\lib\msvc\vc10;
C:\oracle\instantclient_12_1_64;
before C:\oracle\product\11.2.0\client_1\bin
ie your path should be like this
C:\oracle\instantclient_12_1_64\sdk\lib\msvc\vc10;C:\oracle\instantclient_12_1_64;C:\oracle\product\11.2.0\client_1\bin
Just Verified the same softwares/steps works on windows server 2008 r2
Wednesday, August 13, 2014
EJB3.1 - EJB on Websphere & Client on Remote PC with J2SE
Purpose: I was looking for a simple hello world ejb (ejb3.1) with remote interface, that i can deploy on websphere application server and use it from the remote j2se client.
I was not able to find this kind of sample readily available.
So here is the HelloWorld EJB(3.1) and its client.
1) Create HelloWorld EJB
1.1) Using RAD (/eclipse) Create a new JavaEE > Enterprise Application Project.
Project Name = TestEjb, Under java ee module dependency, check the check box for ejb module.
The project Structure should look like below.
Code for TestEJBRemote.java
---------------------------------------------------------------------------------------------------------------------
package ejb31.test;
import javax.ejb.Remote;
@Remote
public interface TestEJBRemote {
public String sayHello(String value);
}
---------------------------------------------------------------------------------------------------------------------
Code for TestEJBRemote.java
---------------------------------------------------------------------------------------------------------------------
package ejb31.test;
import javax.ejb.Stateless;
@Stateless
public class TestEJB implements TestEJBRemote {
public TestEJB() {
// TODO Auto-generated constructor stub
}
@Override
public String sayHello(String yourValue) {
System.out.println("got your " + yourValue);
return "hello " + System.currentTimeMillis() + " - " + yourValue;
}
}
---------------------------------------------------------------------------------------------------------------------
Code for ejb-jar.xml
---------------------------------------------------------------------------------------------------------------------
<?xml version="1.0" encoding="UTF-8"?>
<ejb-jar xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/ejb-jar_3_1.xsd" version="3.1">
<module-name>TestEjbEJB</module-name>
<display-name>TestEjbEJB</display-name>
</ejb-jar>
---------------------------------------------------------------------------------------------------------------------
Code for ibm-ejb-jar-bnd.xml
--------------------------------------------------------------------------------------------------------------------- <?xml version="1.0" encoding="UTF-8"?>
<ejb-jar-bnd
xmlns="http://websphere.ibm.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://websphere.ibm.com/xml/ns/javaee http://websphere.ibm.com/xml/ns/javaee/ibm-ejb-jar-bnd_1_2.xsd"
version="1.2">
<session name="TestEJB"></session>
</ejb-jar-bnd>
---------------------------------------------------------------------------------------------------------------------
Code for ibm-ejb-jar-ext.xml
---------------------------------------------------------------------------------------------------------------------
<?xml version="1.0" encoding="UTF-8"?>
<ejb-jar-ext
xmlns="http://websphere.ibm.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://websphere.ibm.com/xml/ns/javaee http://websphere.ibm.com/xml/ns/javaee/ibm-ejb-jar-ext_1_1.xsd"
version="1.1">
</ejb-jar-ext>
---------------------------------------------------------------------------------------------------------------------
Start the Application server (WAS 8.5 / 8) and deploy to the server.
2) Create the EJB Client.
2.1) Right click on TestEjbEJB and click on Export. Export it as EJB JAR File.
The jar file get exported at location c:/temp.
2.2) Now run the IBM createEJBStubs tool located under AppServer/bin directory.
(run from c:/temp)
C:\IBM\WebSphere85\AppServer_1\bin\createEJBStubs.bat TestEjbEJB.jar -newfile TestEjbEJBClient.jar -quiet
You can check the TestEjbEJBClient.jar - there would be a stub produced by the name of _TestEJBRemote_Stub.class
3) Create the Java Client Project.
Dependency needed
3.1) Create new Java Project TestEJBClient, as below.
Code for TestMain.java
---------------------------------------------------------------------------------------------------------------------
package test;
import java.util.Hashtable;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import ejb31.test.TestEJBRemote;
public class TestMain {
Context ctx = null;
public static void main(String args[]) {
TestMain t = new TestMain();
t.test();
}
public void test() {
Hashtable env = new Hashtable();
env.put(Context.INITIAL_CONTEXT_FACTORY,"com.ibm.websphere.naming.WsnInitialContextFactory");
env.put(Context.PROVIDER_URL,"iiop://localhost:2809");
try {
ctx = new InitialContext(env);
String jndiURL = "ejb31.test.TestEJBRemote";
TestEJBRemote remote = (TestEJBRemote) ctx.lookup(jndiURL);
System.out.println(remote.sayHello("did you got me"));
} catch (NamingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}catch(Exception e) {
e.printStackTrace();
}
}
}
---------------------------------------------------------------------------------------------------------------------
I was not able to find this kind of sample readily available.
So here is the HelloWorld EJB(3.1) and its client.
1) Create HelloWorld EJB
1.1) Using RAD (/eclipse) Create a new JavaEE > Enterprise Application Project.
Project Name = TestEjb, Under java ee module dependency, check the check box for ejb module.
The project Structure should look like below.
Code for TestEJBRemote.java
---------------------------------------------------------------------------------------------------------------------
package ejb31.test;
import javax.ejb.Remote;
@Remote
public interface TestEJBRemote {
public String sayHello(String value);
}
---------------------------------------------------------------------------------------------------------------------
Code for TestEJBRemote.java
---------------------------------------------------------------------------------------------------------------------
package ejb31.test;
import javax.ejb.Stateless;
@Stateless
public class TestEJB implements TestEJBRemote {
public TestEJB() {
// TODO Auto-generated constructor stub
}
@Override
public String sayHello(String yourValue) {
System.out.println("got your " + yourValue);
return "hello " + System.currentTimeMillis() + " - " + yourValue;
}
}
---------------------------------------------------------------------------------------------------------------------
Code for ejb-jar.xml
---------------------------------------------------------------------------------------------------------------------
<?xml version="1.0" encoding="UTF-8"?>
<ejb-jar xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/ejb-jar_3_1.xsd" version="3.1">
<module-name>TestEjbEJB</module-name>
<display-name>TestEjbEJB</display-name>
</ejb-jar>
---------------------------------------------------------------------------------------------------------------------
Code for ibm-ejb-jar-bnd.xml
--------------------------------------------------------------------------------------------------------------------- <?xml version="1.0" encoding="UTF-8"?>
<ejb-jar-bnd
xmlns="http://websphere.ibm.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://websphere.ibm.com/xml/ns/javaee http://websphere.ibm.com/xml/ns/javaee/ibm-ejb-jar-bnd_1_2.xsd"
version="1.2">
<session name="TestEJB"></session>
</ejb-jar-bnd>
---------------------------------------------------------------------------------------------------------------------
Code for ibm-ejb-jar-ext.xml
---------------------------------------------------------------------------------------------------------------------
<?xml version="1.0" encoding="UTF-8"?>
<ejb-jar-ext
xmlns="http://websphere.ibm.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://websphere.ibm.com/xml/ns/javaee http://websphere.ibm.com/xml/ns/javaee/ibm-ejb-jar-ext_1_1.xsd"
version="1.1">
</ejb-jar-ext>
---------------------------------------------------------------------------------------------------------------------
Start the Application server (WAS 8.5 / 8) and deploy to the server.
2) Create the EJB Client.
2.1) Right click on TestEjbEJB and click on Export. Export it as EJB JAR File.
The jar file get exported at location c:/temp.
2.2) Now run the IBM createEJBStubs tool located under AppServer/bin directory.
(run from c:/temp)
C:\IBM\WebSphere85\AppServer_1\bin\createEJBStubs.bat TestEjbEJB.jar -newfile TestEjbEJBClient.jar -quiet
You can check the TestEjbEJBClient.jar - there would be a stub produced by the name of _TestEJBRemote_Stub.class
3) Create the Java Client Project.
Dependency needed
3.1) Create new Java Project TestEJBClient, as below.
Code for TestMain.java
---------------------------------------------------------------------------------------------------------------------
package test;
import java.util.Hashtable;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import ejb31.test.TestEJBRemote;
public class TestMain {
Context ctx = null;
public static void main(String args[]) {
TestMain t = new TestMain();
t.test();
}
public void test() {
Hashtable env = new Hashtable();
env.put(Context.INITIAL_CONTEXT_FACTORY,"com.ibm.websphere.naming.WsnInitialContextFactory");
env.put(Context.PROVIDER_URL,"iiop://localhost:2809");
try {
ctx = new InitialContext(env);
String jndiURL = "ejb31.test.TestEJBRemote";
TestEJBRemote remote = (TestEJBRemote) ctx.lookup(jndiURL);
System.out.println(remote.sayHello("did you got me"));
} catch (NamingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}catch(Exception e) {
e.printStackTrace();
}
}
}
---------------------------------------------------------------------------------------------------------------------
Thursday, May 1, 2014
Head FIrst JQuery by Ryan Benedetti and Ronan Cranley, O’Reilly Media
First of all big thanks to the "Head First" series designers who gave a very good thought of how the book need to be organised, the section "How to use this book" tells about all the book, the software required, examples location and how to set up the developers workspace.
After giving a introduction the author goes over the various indepth of JQuery like selectors, methods, events & function, and keeps going on to the deeper part where jquery is used the most - Web Page manipulations, Effects, Animation, Custom Functions, Ajax and JSON.
The last two chapters are dedicated to jquery widgets aka JQuery UI and indepth of JQuery API and objects.
Would have some more explanation of JSON and how that can benefit the data driven pages.
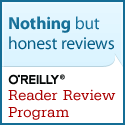
Thursday, September 19, 2013
Websphere 8.5 Mediation Handler
Application Server: Websphere 8.5.5, RAD8.5,
Application: SampleMediation
What the application do: the application mediates any message coming on one destination to the other specified destination
Create Application Structure as below
>SampleMediation (EAr Project)
>SampleMediationEJB (ejb project)
>Mediation (java project)
Under Mediation Add a sample mediation handler as below:
public class FirstMediationHandler implements MediationHandler {
private String outDestination = "out.queue";
@Override
public boolean handle(MessageContext msgCtx) throws MessageContextException {
System.out.println("--incoming--");
SIMessageContext siMsgCtx = (SIMessageContext) msgCtx;
SIMessage originalMsg = siMsgCtx.getSIMessage();
//you do loads of thing, like transformation
List frp = new ArrayList(1);
SIDestinationAddress dest = SIDestinationAddressFactory.getInstance().createSIDestinationAddress(outDestination, false);
frp.add(dest);
originalMsg.setForwardRoutingPath(frp);
System.out.println("--routed--");
return true;
}
}
>SampleMediationEJB (ejb project), add ejb-jar.xml and ws-handler.xmi
ejb-jar.xml should have following<session id="FirstMediationHandler">
<ejb-name>FirstMediationHandler</ejb-name>
<local-home>com.ibm.websphere.sib.mediation.handler.ejb.GenericEJBMediationHandlerLocalHome</local-home>
<local>com.ibm.websphere.sib.mediation.handler.ejb.GenericEJBMediationHandlerLocal</local>
<ejb-class>com.ibm.websphere.sib.mediation.handler.ejb.GenericEJBMediationHandlerBean</ejb-class>
<session-type>Stateless</session-type>
<transaction-type>Container</transaction-type>
<env-entry>
<env-entry-name>mediation/MediationHandlerClass</env-entry-name>
<env-entry-type>java.lang.String</env-entry-type>
<env-entry-value>com.vsp.test.FirstMediationHandler</env-entry-value>
</env-entry>
</session>
ws-handler.xmi should have the following
<handler:EJBHandlerDD xmi:id="EJBHandlerDD1" name="FirstMediationHandler" description="" critical="true">
<lists xmi:id="HandlerListRef1" listName="first_mediation" description="mediates from vcin_in to cch_in" sequence="1"/>
<ejb xmi:type="ejb:Session" href="META-INF/ejb-jar.xml#FirstMediationHandler"/>
</handler:EJBHandlerDD>
Thats it build the ear and do the setup as below.
Setup:
1) Create a local bus - bus1,
2) Create two destinations - out.queue and in.queue
3) Configure queues
- jms/out.queue for destination out.queue
- jms/in.queue for destination in.queue
4) Deploy the SampleMediation.ear
5) Create new Mediation in websphere,
Buses > bus1 > Mediations - create new, following values are entered
name = first_mediation
Handler list name = first_mediation
6) Mediate the destination
Go to Buses > bus1 > Destinations > in.queue select it by clicking the checkbox
and click the Mediate button on the top
7) Select Mediation
The mediation to apply to this destination = first_mediation
8) The mediation would be up and working and any message coming on the in.queue is routed to out.queue
Wednesday, May 29, 2013
Websphere 8.5 + EJB3.1 Interpreting error logs
Injection (@Inject) would not work. Following error is reported.
000000bc InjectInjecti E CWOWB0102E: A JCDI error has occurred: JCDI runtime cannot resolve @Inject java.lang.reflect
solution: Went and look in to ffdc logs and found that Entities were not correctly created and hence the whole ORM was failing to load up. fixed the ORM objects (mapping etc) and issue get resolved
000000bc InjectInjecti E CWOWB0102E: A JCDI error has occurred: JCDI runtime cannot resolve @Inject java.lang.reflect
solution: Went and look in to ffdc logs and found that Entities were not correctly created and hence the whole ORM was failing to load up. fixed the ORM objects (mapping etc) and issue get resolved
Friday, March 29, 2013
Minimize the Carbon foot print.
Minimize the Carbon foot print.
It would be very hard to conveince the goverment to lay down the rules to protect the envrioment, as this would lead this article to a wrong directions. But we as grown-up always worry about future of our children and grand-childrens. We want to save money for there education, save real-estate or property for there living, save on retirement but have ever thought of saving oxygen or this environment on which we depend. Have we ever made a small decission that i would not use plastic - no matter what happens. Frankly tell me what are the implications that we stop using plastic bottles, plastic bags, plastic boxes, - this would not make lay-offs but this would rather increase the job market as the vary company producing this would have to invest in to producing degradable bags, boxes, bottles - more jobs and less danger to environment and our future.
I totally agreee that taking plastic off completely is not possible, but we can minimize the use.
Here are some way to do that
1) after you done grocerry shopping please ask them to use Paper Bags - as the bags are generally thrown away once the Items reach home.
2) Generally carry a reusable water bottle - saying no to “Plastic bottled Water” but we can refill it using the “Big Water Dispenser” - every thing supplied from the same company who makes the “plastic bottled water”.
3) Please say no to StyroFoam (Polystyrene) - cups or glasses - this Styrofoam is very hard material and would not degrade easily. Please search online to findout why i am asking not to use this. Let companies making them innovate themselves as one Water-company supplying bottled water to us already started supplying “small - paper cups” which is used to drink water and then discard.
The cups - glasses are used for hot/cold beverages,water, ice etc etc, but one can have there own “Mug” and reduce it use.
4) we can use Paper plates & bowls instead of styrofoam plates & bowls.
5) Recycle - yes i do agree that in gettogether, parties,picnics we cannot do it with out plastics forks, knifes, spoons but please recycle them. Ask the people to seperate them out when discarding.
To minimize cabron footprint - heres a list of things you can do
Subscribe to:
Posts (Atom)